How to Fix Docker Bypassing Firewall: A Complete Guide
Learn how you can fix the docker security issue that is bypassing the firewall
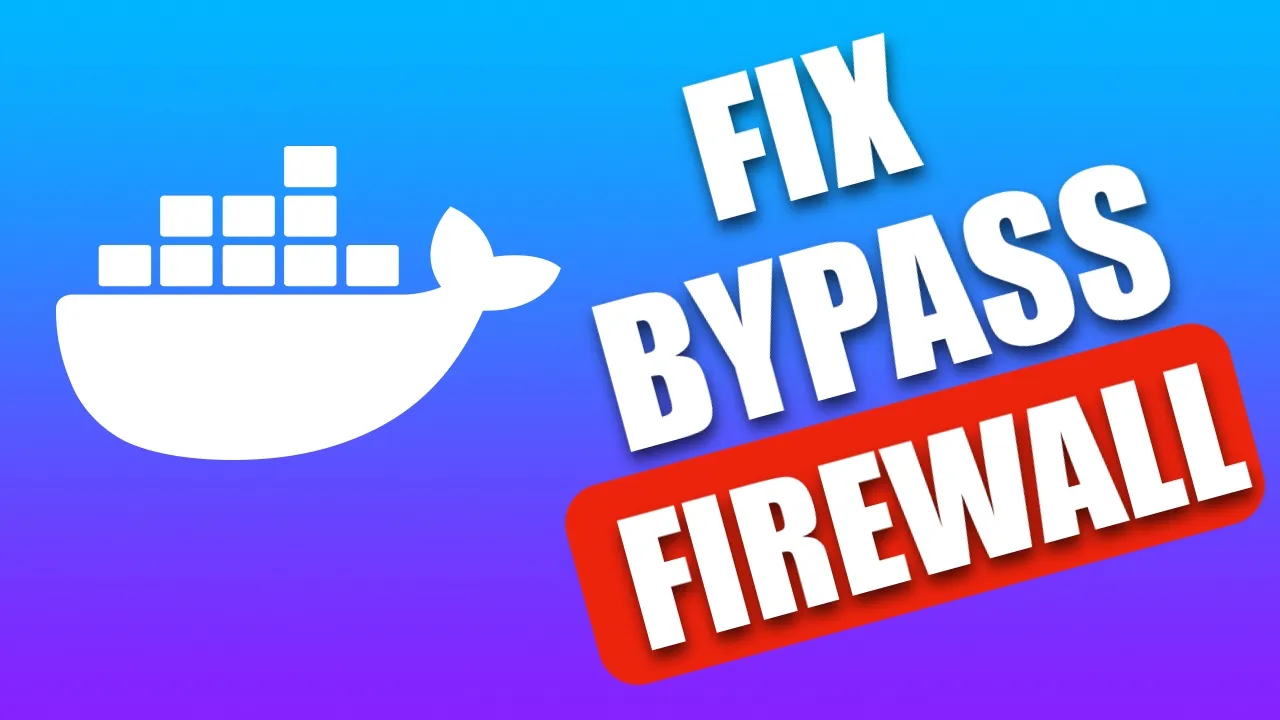
I recently discovered something quite surprising while setting up Docker containers on my VPS - Docker can actually bypass your system’s firewall rules! If you’re managing your own servers and running Docker containers, this is something you definitely need to know about.
Understanding the Problem: Why Docker Bypasses the Firewall
Here’s what I learned: When you set up Docker on your system, it creates its own networking setup that works a bit differently than you might expect. Let me break down what’s actually happening behind the scenes.
Docker’s default behavior prioritizes ease of use for container networking, which involves automatically setting up these firewall rules. While this makes container networking more straightforward and user-friendly, it can inadvertently create security vulnerabilities if not managed properly. Docker needs this level of network control to efficiently handle inter-container communication and external access, but it’s crucial to understand and manage these automatic configurations.
By default, Docker sets up something called a bridge network (usually named docker0
). Think of it as Docker creating its own little private network inside your system. When you run a container with a port mapping like this:
services:
myapp:
image: nginx
ports:
- "8080:80"
If you are interested to see some free cool open source self hosted apps you can check toolhunt.net self hosted section.
You might think you’re just opening port 8080 on localhost, but what’s actually happening is more complex. Docker is binding to 0.0.0.0:8080
, which means it’s accessible from anywhere, not just your local machine. This is similar to the issue I encountered when setting up containers for my home server.
The real kicker is that Docker modifies your iptables (the Linux firewall) directly to make this work. It adds its own rules before your regular firewall rules, which means those carefully crafted firewall configurations you set up might not be doing what you expect for your Docker containers.
Here’s a quick way to see this in action. Try running:
sudo iptables -L -n -v | grep DOCKER
You’ll probably see several rules that Docker has added to your firewall configuration - these are what can bypass your regular firewall rules.
This behavior is by design - Docker needs to efficiently manage container networking and provide seamless communication between containers and the outside world. However, this convenience comes with security implications that need to be carefully considered and managed. In the following sections, I’ll show you exactly how to fix this and secure your Docker containers properly while maintaining the functionality you need.
Solutions: Configuring the Firewall to Control Docker Traffic
After discovering this security concern, I’ve found several effective ways to handle it. Let me share the most practical solutions I’ve implemented and tested.
1. Using Localhost Binding (The Simplest Fix)
The easiest solution I’ve found is to explicitly bind containers to localhost. Instead of letting Docker bind to all interfaces (0.0.0.0
), we can force it to only listen on 127.0.0.1
. Here’s how to do it in your docker-compose.yml:
services:
myapp:
image: nginx
ports:
- "127.0.0.1:8080:80"
While this is the simplest solution, it does have limitations. If you need other services on the same host to access the container, or if you plan to expose the service directly without a reverse proxy, this method won’t work. In such cases, you’ll need to consider alternative approaches like those discussed below.
2. Using a Cloud Firewall
One approach I’ve come to really appreciate is using a cloud firewall instead of relying solely on the VPS firewall. Cloud providers offer various firewall solutions:
- AWS Security Groups: Configure inbound/outbound rules at the instance level
- Google Cloud Firewall Rules: Network-level filtering with tag-based rules
- DigitalOcean Cloud Firewalls: Similar to AWS security groups
- Hetzner Cloud Firewall: Network-level filtering before traffic reaches your VPS
These firewalls operate at the network level, meaning they can’t be bypassed by Docker’s internal networking configuration.
The advantage here is that these firewalls can’t be bypassed by Docker’s networking tricks because they operate at a higher level. Here’s what I typically do:
- Allow only necessary ports (usually 80, 443 for web traffic, and 22 for SSH)
- Block all other incoming traffic
- Configure specific rules for any additional services
3. Using Traefik as a Reverse Proxy
I’ve found that using Traefik as a reverse proxy is one of the most elegant solutions. Here’s a basic setup:
version: '3'
services:
traefik:
image: traefik:v2.10
command:
- "--providers.docker=true"
- "--api.dashboard=true"
- "--api.authentication.basic.users=admin:$$apr1$$xyz123$$" # Use htpasswd to generate
- "--certificatesresolvers.letsencrypt.acme.tlschallenge=true"
- "[email protected]"
ports:
- "127.0.0.1:80:80"
volumes:
- /var/run/docker.sock:/var/run/docker.sock:ro
labels:
- "traefik.enable=true"
- "traefik.http.routers.dashboard.middlewares=auth@file"
myapp:
image: nginx
labels:
- "traefik.enable=true"
- "traefik.http.routers.myapp.rule=Host(`your-domain.com`)"
# Notice: no ports exposed directly!
When using Traefik, ensure you secure the proxy itself:
- Enable HTTPS with automatic Let’s Encrypt certificates
- Add authentication for the Traefik dashboard
- Use secure headers and middleware
- Regularly update Traefik to the latest version
This setup is particularly secure because:
- Only Traefik’s ports are exposed, and they’re bound to localhost
- Other containers don’t expose ports directly
- All traffic is routed through Traefik
- Built-in SSL/TLS support with automatic certificate management
4. Advanced Firewall Configurations: Working with iptables and UFW
After setting up the basic protections, I learned there are more sophisticated ways to control Docker’s network access. Let me share how to handle this using different firewall tools.
Working with iptables
The DOCKER-USER chain is special because Docker specifically avoids modifying rules within this chain, making it a safe place for administrators to add their custom firewall rules that will persist. This chain is processed before Docker’s automatic rules, giving you precise control over incoming container traffic.
Here’s how I set it up:
# Allow established connections
sudo iptables -A DOCKER-USER -i eth0 -j ACCEPT -m conntrack --ctstate ESTABLISHED,RELATED
# Allow specific IPs (replace with your trusted IPs)
sudo iptables -A DOCKER-USER -i eth0 -s 203.0.113.1 -j ACCEPT
# Drop everything else
sudo iptables -A DOCKER-USER -i eth0 -j DROP
To make these rules permanent (survive reboots), I use:
sudo netfilter-persistent save
sudo netfilter-persistent reload
Working with firewalld
‘firewalld’ uses the concept of “zones” to represent different network environments and applies different rules based on the active zone. To effectively manage Docker traffic, you can create specific zones for your Docker interfaces and networks.
Here’s how you can configure firewalld to control traffic to Docker networks:
- Create a Zone for Docker:
sudo firewall-cmd --permanent --new-zone=docker
- Bind Docker Interfaces:
# For default docker0 bridge
sudo firewall-cmd --permanent --zone=docker --add-interface=docker0
# For user-defined networks (replace br-xxx with your network interface)
sudo firewall-cmd --permanent --zone=docker --add-interface=br-xxx
- Define Rules within the Docker Zone:
# Allow specific ports
sudo firewall-cmd --permanent --zone=docker --add-port=8080/tcp
# Allow specific services
sudo firewall-cmd --permanent --zone=docker --add-service=http
- Apply and Verify:
# Reload firewalld
sudo firewall-cmd --reload
# Verify configuration
sudo firewall-cmd --zone=docker --list-all
For user-defined Docker networks, you’ll need to:
- Identify the bridge interface name:
docker network ls
docker network inspect network_name | grep "Interface"
- Add the interface to your firewall zone:
sudo firewall-cmd --permanent --zone=docker --add-interface=br-xxxxx
Using UFW (Uncomplicated Firewall)
Setting DEFAULT_FORWARD_POLICY to “ACCEPT” is necessary because Docker requires the ability to forward traffic through its bridge network. This setting allows containers to communicate with the outside world through the host’s network interface. However, this makes it even more important to carefully configure your container-specific rules.
- First, edit
/etc/default/ufw
:
DEFAULT_FORWARD_POLICY="ACCEPT"
- Then, create Docker-specific rules in
/etc/ufw/after.rules
:
# NAT table rules
*nat
:POSTROUTING ACCEPT [0:0]
# Forward traffic through eth0
-A POSTROUTING -s 172.17.0.0/16 ! -o docker0 -j MASQUERADE
COMMIT
# Don't delete these required lines
*filter
:ufw-user-forward - [0:0]
:ufw-docker-logging-deny - [0:0]
:DOCKER-USER - [0:0]
# Docker user chain
-A DOCKER-USER -j RETURN -s 10.0.0.0/8
-A DOCKER-USER -j RETURN -s 172.16.0.0/12
-A DOCKER-USER -j RETURN -s 192.168.0.0/16
-A DOCKER-USER -j ufw-user-forward
-A DOCKER-USER -j DROP
COMMIT
- Apply the changes:
sudo ufw reload
Using Docker Compose Networks
One thing that really helped me secure my containers was using custom networks in Docker Compose. Understanding the difference between internal and external networks is crucial for security:
-
Internal networks (
internal: true
):- Completely isolated from external networks
- Containers can’t make outbound connections
- Perfect for sensitive services like databases
- Only containers within the same internal network can communicate
-
External networks (
internal: false
):- Allow containers to access the internet
- Can be accessed from outside the Docker host
- Suitable for public-facing services
- Need careful firewall configuration
Here’s an example of how I organize my containers with network isolation:
version: '3'
networks:
frontend:
internal: false # Allows external access
backend:
internal: true # Completely isolated from external access
services:
web:
image: nginx
networks:
- frontend
ports:
- "127.0.0.1:8080:80"
security_opt: # Advanced security options
- no-new-privileges:true
api:
image: node
networks:
- frontend
- backend
depends_on:
- database
database:
image: mysql
networks:
- backend # Only connected to internal network
environment:
MYSQL_ROOT_PASSWORD_FILE: /run/secrets/db_root_password
secrets:
- db_root_password
security_opt:
- no-new-privileges:true
secrets:
db_root_password:
file: ./secrets/db_password.txt
This setup ensures that:
- The database is completely isolated from external access
- The API service can communicate with both web and database
- All external access must go through localhost
- Additional security measures like secrets and security options are implemented
For more complex setups, you might want to implement additional security measures:
- Network Segmentation:
networks:
frontend:
internal: false
ipam:
config:
- subnet: 172.20.0.0/24
backend:
internal: true
ipam:
config:
- subnet: 172.20.1.0/24
- Network Encryption (for swarm mode):
networks:
backend:
driver: overlay
driver_opts:
encrypted: "true"
- Rate Limiting with Traefik:
services:
web:
labels:
- "traefik.http.middlewares.ratelimit.ratelimit.average=100"
- "traefik.http.middlewares.ratelimit.ratelimit.burst=50"
- Container Resource Limits:
services:
web:
deploy:
resources:
limits:
cpus: '0.50'
memory: 512M
reservations:
cpus: '0.25'
memory: 256M
When managing these networks, it’s important to regularly audit their configuration:
# List all networks
docker network ls
# Inspect network configuration
docker network inspect frontend
# Check container connectivity
docker network connect test-network container-name
# Monitor network usage
docker stats --format "table {{.Name}}\t{{.NetIO}}"
Remember to periodically clean up unused networks:
# Remove unused networks
docker network prune
# Remove specific network
docker network rm network-name
When managing multiple containers, I also find it helpful to use Docker Compose secrets for additional security.
Conclusion: Keeping Your Docker Containers Secure
After spending considerable time learning about Docker’s networking quirks and implementing various security measures, I’ve realized that securing Docker containers isn’t as straightforward as it might seem at first. However, it’s definitely manageable once you understand what’s happening under the hood.
Here’s what I’ve learned to be the most important takeaways:
-
Always Start with the Basics
- Bind containers to localhost when possible
- Use reverse proxies like Traefik for external access
- Regularly clean up unused Docker resources to maintain a clean, secure environment
-
Layer Your Security
- Don’t rely on just one security measure
- Combine host firewall rules with Docker network isolation
- Consider using cloud firewalls as an additional security layer
-
Best Practices I Now Follow
- Regularly audit exposed ports using
docker ps
- Keep Docker and containers updated
- Use the principle of least privilege when managing Docker users
- Document all Docker commands and configurations for easy maintenance
- Regularly audit exposed ports using
Remember, security is an ongoing process. I recommend regularly reviewing your Docker networking setup and firewall rules to ensure they’re still appropriate for your needs.
A quick checklist I use for any new Docker deployment:
# Check exposed ports
docker ps
# Review network configurations
docker network ls
# Verify firewall rules
sudo iptables -L -n -v | grep DOCKER
# Check Docker daemon configuration
docker info
The most important thing I’ve learned is that while Docker’s default networking behavior might seem concerning at first, there are plenty of tools and techniques available to secure it properly. The key is understanding how these pieces fit together and implementing the right combination of security measures for your specific needs.
If you’re just getting started with Docker, you might want to check out how to copy multiple files efficiently in Dockerfiles and other basic Docker concepts before diving deep into security configurations.
By following these guidelines and regularly reviewing your security setup, you can run Docker containers confidently while maintaining proper security controls. Remember, security isn’t about implementing every possible measure, but about choosing the right combination of tools and practices that work for your specific situation.
Related Posts
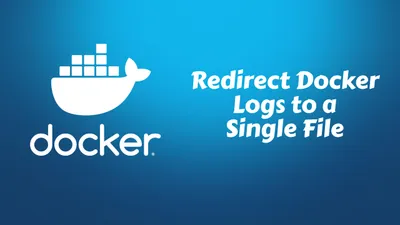
How to Redirect Docker Logs to a Single File
Learn how to Redirect Docker Logs to a Single File to make your work easier
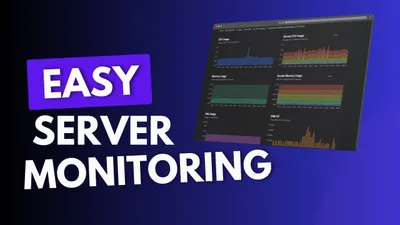
How To Monitor Server and Docker Resources:CPU,Memory...
Learn how you can monitor sever resources like CPU, memory, disk space including Docker containers and get notified in case something happens.
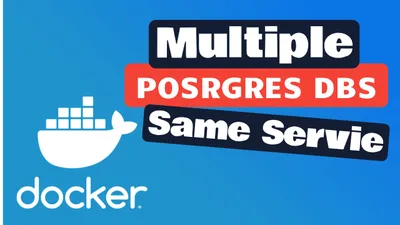
Multiple PostgreSQL Databases in ONE Service: THE Docker Compose WAY!
Master multiple PostgreSQL databases effortlessly! Discover how Docker Compose simplifies your setup. Don't miss out – transform your workflow NOW!