Building an AI Agent with Agno and Context7 MCP
How to connect Agno to Context7's MCP server for accessing up-to-date library documentation and code snippets through AI agents
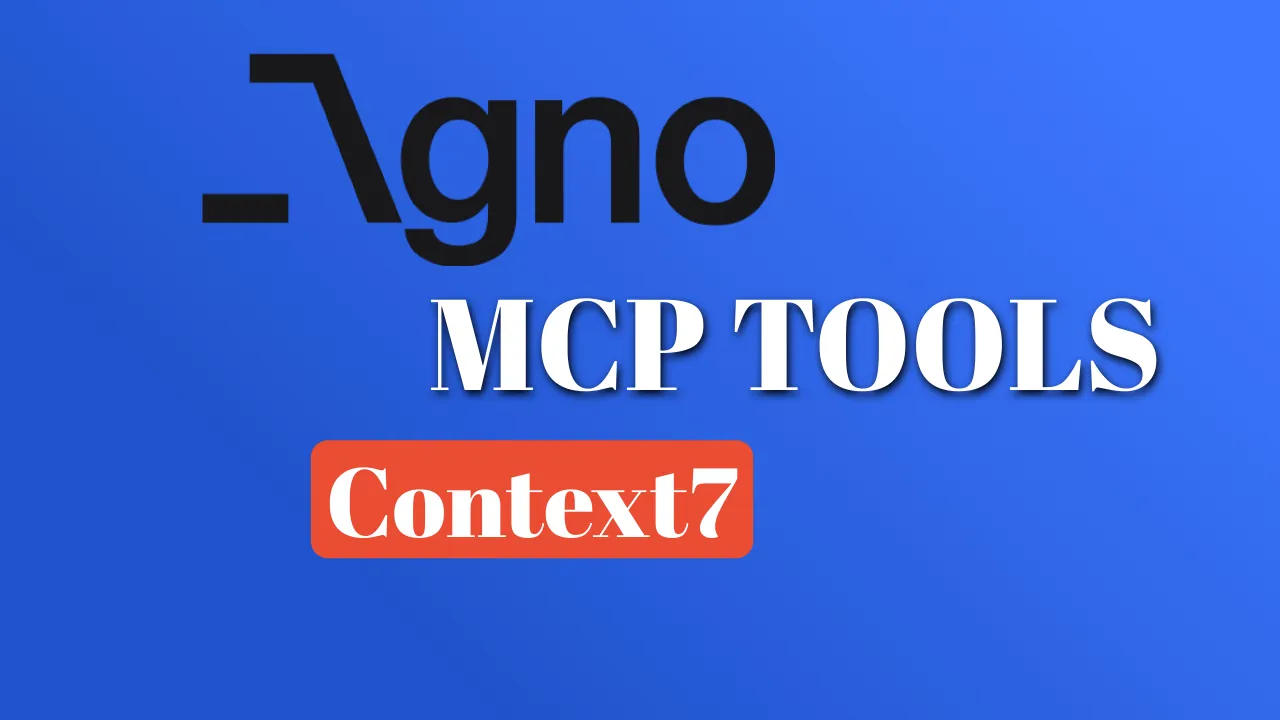
Agno Agents Tutorials
Part 4 of 4
Table of Contents
- Why Use Context7 with Agno?
- Prerequisites
- Step 1: Setting Up Your Environment
- Step 2: Configure MCP to Use Context7
- Step 3: Understanding Context7 MCP Integration
- Step 4: Creating an AI Agent Using Context7
- Step 5: Complete Async Example with User Interaction
- Step 6: Testing the Agent
- Step 7: Best Practices and Optimization
- Other MCP tools and AGNO Toolkit
- Final Thoughts
In the rapidly evolving world of software development, staying updated with the latest library documentation and code examples is crucial. By integrating Agno, a powerful AI agent framework, with Context7’s MCP (Managed Context Provider) server, you can create intelligent AI agents capable of querying real-time documentation and code snippets for any software library. This guide provides a detailed, step-by-step process to set up and deploy such an AI agent, complete with enhanced explanations, best practices, and a robust example.
Why Use Context7 with Agno?
Context7 is a specialized service that delivers up-to-date documentation and code examples optimized for large language models (LLMs) and AI-driven code editors. When paired with Agno, an AI agent framework designed for extensibility and tool integration, you can build agents that:
- Dynamically fetch the latest API documentation for any library.
- Retrieve relevant code snippets to demonstrate library usage.
- Answer complex queries about library features, usage patterns, or troubleshooting.
- Streamline developer workflows by providing instant, context-aware assistance for coding, debugging, and learning.
This integration is ideal for developers, educators, and teams looking to enhance productivity and reduce the time spent searching for documentation.
Prerequisites
Before diving into the setup, ensure you have the following:
- Python 3.12+ installed with uv
- Node.js and npm for running the Context7 MCP server.
- An OpenAI API key for powering the AI model.
- Basic familiarity with Python, asynchronous programming, and command-line tools.
Step 1: Setting Up Your Environment
Install Required Packages
Install the necessary Python packages using pip:
uv add agno openai mcp
Additionally, ensure you have Node.js installed to run the Context7 MCP server. You can verify this by running:
node --version
npm --version
Set Environment Variables
Set your OpenAI API key as an environment variable to authenticate API requests:
-
Linux/macOS:
export OPENAI_API_KEY='your-openai-api-key'
-
Windows (Command Prompt):
set OPENAI_API_KEY=your-openai-api-key
Alternatively, you can set the variable in your Python script or a .env
file using a library like python-dotenv
.
Best Practice: Store sensitive keys in environment variables or a secure vault to prevent accidental exposure in code.
Step 2: Configure MCP to Use Context7
The Context7 MCP server is launched using a Node.js command. Define the server configuration in your Python code as follows:
mcp_servers_config = {
"context7": {
"command": "npx",
"args": ["-y", "@upstash/context7-mcp@latest"]
}
}
This configuration specifies that the npx
command will run the latest version of the Context7 MCP server. The -y
flag ensures automatic confirmation for npm prompts.
Note: Ensure you have an active internet connection, as npx
downloads the latest @upstash/context7-mcp
package on demand.
Step 3: Understanding Context7 MCP Integration
The Context7 MCP server acts as a bridge between your AI agent and Context7’s vast repository of library documentation and code snippets. Key capabilities include:
- Dynamic Search: Query library APIs and documentation in real time.
- Code Snippet Retrieval: Fetch practical, up-to-date code examples for specific library features.
- Natural Language Queries: Allow the agent to interpret user questions and map them to relevant documentation.
- Scalability: Handle queries for thousands of libraries across programming languages and frameworks.
By integrating with Agno, the agent can leverage these capabilities through structured tool calls, making it a powerful assistant for developers.
Step 4: Creating an AI Agent Using Context7
To create the AI agent, you need to:
- Initialize the MCPTools with the Context7 command.
- Set up an Agno agent with an OpenAIChat model (e.g., GPT-4.1-mini).
- Provide detailed instructions for querying Context7 MCP.
Here’s an example of the initialization code:
from agno.tools.mcp import MCPTools
from agno.agent import Agent
from agno.models.openai import OpenAIChat
async def main():
# Context7 MCP command
command = "npx -y @upstash/context7-mcp@latest"
async with MCPTools(command, args) as mcp_tools:
agent = Agent(
name="Agno Context7 Doc Agent",
role="An AI agent that provides up-to-date library documentation and code snippets using Context7 MCP.",
model=OpenAIChat(id="gpt-4.1-mini"),
tools=[mcp_tools],
instructions='''
You are an AI assistant that helps users find up-to-date library documentation and code snippets via Context7 MCP.
When a user asks for documentation:
1. Identify the first word or phrase in the user's request as the library name (e.g., "agno" in "agno mcp tools").
2. Use the `resolve-library-id` tool with the identified library name to get the Context7-compatible ID.
3. Consider the rest of the user's request *after* the library name as the topic for the `get-library-docs` tool (e.g., "mcp tools" in "agno mcp tools").
4. Use the `get-library-docs` tool with the resolved library ID and the topic identified in step 3.
5. Use the default token limit of 5000 unless the user specifies a different number.
6. Return the documentation and code snippets in a clear and concise format based on the tool results.
When constructing MCP tool calls:
- `resolve-library-id` requires a `libraryName` string.
- `get-library-docs` requires a `context7CompatibleLibraryID` string.
- Include the `topic` parameter for `get-library-docs` if a topic was identified.
- Optionally include the `tokens` parameter for `get-library-docs` if specified by the user.
Always explain your reasoning and show tool call results in a user-friendly format.
**Example Interaction**:
User: "requests how to make a GET request"
1. Library name: "requests"
2. Topic: "how to make a GET request"
3. Call `resolve-library-id` with `libraryName="requests"`
4. Call `get-library-docs` with the resolved ID and `topic="how to make a GET request"`
5. Format and return the results
''',
show_tool_calls=True,
add_state_in_messages=True,
markdown=True
)
# Interaction loop will be added in the next step
Key Configuration Details
- Model: The
OpenAIChat(id="gpt-4.1-mini")
specifies a lightweight, efficient model. You can upgrade to a more powerful model (e.g.,gpt-4.1
) for complex queries. - Tools: The
MCPTools
instance connects the agent to the Context7 MCP server. - Instructions: The detailed instructions ensure the agent correctly interprets user queries and uses the MCP tools effectively.
- Markdown: Enabling
markdown=True
ensures formatted, readable output.
Step 5: Complete Async Example with User Interaction
Below is the complete Python script that sets up the environment, creates the agent, and implements an interactive loop for user queries:
import os
import asyncio
from agno.tools.mcp import MCPTools
from agno.agent import Agent
from agno.models.openai import OpenAIChat
if "OPENAI_API_KEY" not in os.environ:
raise ValueError("OPENAI_API_KEY environment variable is not set.")
def print_welcome():
print('''
Agno Context7 Documentation Agent
---------------------------------
Ask questions about libraries or functions.
Type 'exit' or 'quit' to stop.
''')
async def main():
print_welcome()
command = "npx -y @upstash/context7-mcp@latest"
async with MCPTools(command) as mcp_tools:
agent = Agent(
name="Agno Context7 Doc Agent",
role="An AI assistant that fetches up-to-date docs and code snippets using Context7 MCP.",
model=OpenAIChat(id="gpt-4.1-mini"),
tools=[mcp_tools],
instructions='''
You are an AI assistant that helps users find up-to-date library documentation and code snippets via Context7 MCP.
When a user asks for documentation:
1. Identify the first word or phrase in the user's request as the library name (e.g., "agno" in "agno mcp tools").
2. Use the `resolve-library-id` tool with the identified library name to get the Context7-compatible ID.
3. Consider the rest of the user's request *after* the library name as the topic for the `get-library-docs` tool (e.g., "mcp tools" in "agno mcp tools").
4. Use the `get-library-docs` tool with the resolved library ID and the topic identified in step 3.
5. Use the default token limit of 5000 unless the user specifies a different number.
6. Return the documentation and code snippets in a clear and concise format based on the tool results.
When constructing MCP tool calls:
- `resolve-library-id` requires a `libraryName` string.
- `get-library-docs` requires a `context7CompatibleLibraryID` string.
- Include the `topic` parameter for `get-library-docs` if a topic was identified.
- Optionally include the `tokens` parameter for `get-library-docs` if specified by the user.
Always explain your reasoning and show tool call results.
''',
show_tool_calls=True,
add_state_in_messages=True,
markdown=True
)
while True:
user_input = input("\nYou: ").strip()
if user_input.lower() in {"exit", "quit"}:
print("Goodbye!")
break
try:
await agent.aprint_response(user_input, stream=True)
except Exception as e:
print(f"\n❌ Error: {str(e)}")
if __name__ == "__main__":
try:
asyncio.run(main())
except KeyboardInterrupt:
print("\nInterrupted. Exiting.")
)
Enhancements in the Example
- Welcome Message: A clear, formatted welcome message guides users on how to interact with the agent.
- Error Handling: Robust exception handling ensures the agent gracefully handles interruptions and errors.
- Streamed Responses: The
stream=True
parameter provides real-time output, improving user experience. - Example Interaction: The instructions include a sample query to clarify how the agent processes requests.
Step 6: Testing the Agent
To test the agent, run the script and try queries like:
requests how to make a GET request
numpy array operations
pandas merge dataframes
The agent will:
- Extract the library name (e.g.,
requests
). - Resolve the Context7-compatible ID using
resolve-library-id
. - Fetch documentation and snippets for the specified topic (e.g.,
how to make a GET request
). - Display the results in a formatted, markdown-compatible output.
Example Output (for requests how to make a GET request
):
**Library**: requests
**Topic**: how to make a GET request
**Documentation**:
The `requests.get()` method is used to send a GET request to a specified URL.
Code Snippet:
import requests
response = requests.get('https://api.example.com/data')
print(response.json())
Tool Calls:
resolve-library-id(libraryName="requests")
->context7_id: requests-py
get-library-docs(context7CompatibleLibraryID="requests-py", topic="how to make a GET request")
Step 7: Best Practices and Optimization
- Token Limits: Adjust the
tokens
parameter inget-library-docs
for large or small documentation needs. - Caching: Implement caching for frequently queried libraries to reduce MCP server calls.
- Error Handling: Log errors to a file or monitoring service for debugging in production.
- Model Selection: Use a more powerful model (e.g.,
gpt-4.1
) for complex queries involving multiple libraries. - Security: Validate user inputs to prevent injection attacks when constructing tool calls.
Other MCP tools and AGNO Toolkit
There is a big number off MCP server that can be used you can create any MCP connection with Agno like for Supabase, Airtable and a lot more, you can find the complete list on Model Context Protocol servers
Agno has his own toolkit with tools that are ready to go and you can easely integrate them. You can see: Agno Toolkit
Final Thoughts
Integrating Context7 MCP with Agno creates a powerful AI agent that delivers real-time, contextually relevant programming documentation and code examples. This setup enhances developer productivity, supports learning, and enables rapid prototyping by providing instant access to the latest library resources.
You can extend this agent by:
- Adding support for multiple MCP servers for different documentation sources.
- Integrating with code editors (e.g., VS Code) for in-editor assistance.
- Building specialized agents for specific domains (e.g., web development, data science).
To package this as a repository, consider creating a GitHub repository with:
- The script as
main.py
. - A
README.md
explaining setup and usage. - A
requirements.txt
for dependencies. - Example queries in a
docs/
folder.
Related Posts
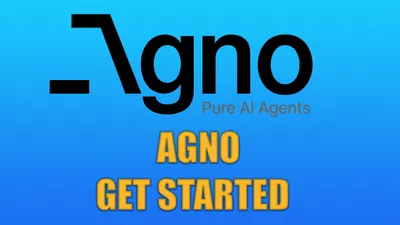
From Zero to Agent Hero: Getting Started with Agno Agents, uv, and a Dash of RAG Magic
Learn how to create powerful AI agents with Agno in minutes! This beginner-friendly guide walks you through setup, basic agents, and advanced features using uv
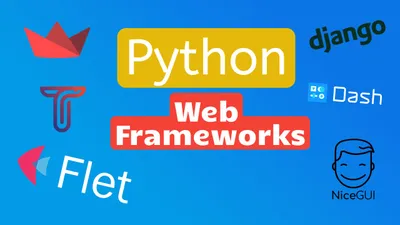
30+ Best Python Web Frameworks for 2024
A complete list with the best Python Web Frameworks for 2024 that you can use to add an interface to your Python applications.
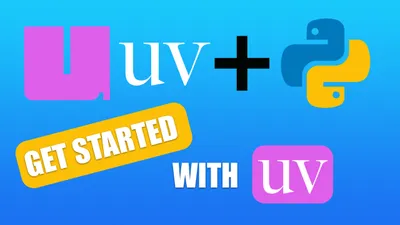
Getting Started with uv: Setting Up Your Python Project in 2025
See how you can get started with uv, a next-generation Python package and project manager written in Rust by the Astral team.