From Zero to Agent Hero: Getting Started with Agno Agents, uv, and a Dash of RAG Magic
Learn how to create powerful AI agents with Agno in minutes! This beginner-friendly guide walks you through setup, basic agents, and advanced features using uv
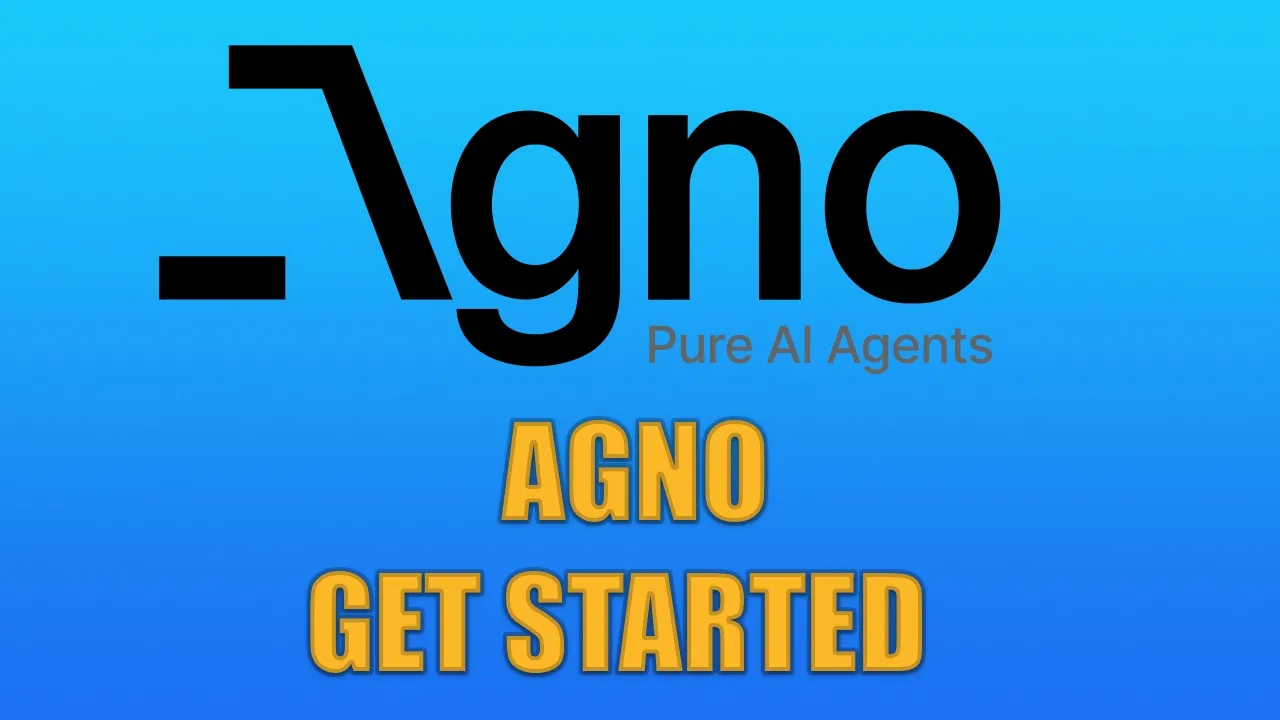
Agno Agents Tutorials
Part 1 of 3
Table of Contents
- Getting Started with Agno Agents
- Step 1: Turbocharge Your Setup with uv—Python Management at Warp Speed
- Step 2: Your First Agno Agent—Simple, Yet Chatty
- Step 3: Adding DuckDuckGo Tools—Your Web-Surfing Sidekick
- Step 4: Memory That Sticks—From Forgetful to Faithful
- Step 5: RAG with LanceDB—Knowledge Is Your Superpower
- Step 6: Team of Two—Chef and Researcher Duo
- Conclusion: Your Agno Journey Takes Flight!
Picture this: it’s March 2025, and you’re ready to unleash an AI sidekick that doesn’t just chat, but searches the web, recalls your undying love for spicy Thai food, and dives into PDFs faster than you can say “where’s my coffee?” Enter Agno, a lightweight, open-source Python library designed to build multimodal AI agents that are as versatile as they are speedy. Paired with uv
, a Rust-powered package manager that leaves pip
in the dust (think 10-100x faster), you’re about to embark on a coding adventure that’s equal parts thrilling and hilarious.
In this guide, we’ll turbocharge your setup with uv
, then craft an Agno agent that evolves from a chatty newbie to a memory-savvy, Retrieval-Augmented Generation (RAG) maestro, powered by tools like LanceDB and DuckDuckGo. We’ll cap it off with a two-agent dream team that collaborates like peanut butter and jelly—or better yet, chicken and galangal. With code snippets, witty asides, and troubleshooting tips, you’ll be laughing your way to AI mastery. Oh, and did we mention Agno’s rumored to be 10,000x faster than LangGraph? Buckle up—we’re testing that claim with a grin!
Getting Started with Agno Agents
Step 1: Turbocharge Your Setup with uv—Python Management at Warp Speed
Before we unleash Agno’s powers, we need a lightning-fast foundation. That’s where uv comes in—a package manager from the Astral crew (the same folks behind ruff
) that’s so quick, it’ll have you wondering why you ever tolerated pip
’s leisurely pace. Let’s get it rolling!
You can check more on how you can get started with uv
Installing uv
For macOS/Linux:
curl -LsSf https://astral.sh/uv/install.sh | sh
For Windows (PowerShell):
irm https://astral.sh/uv/install.ps1 | iex
Check it’s alive:
uv --version # Expect "uv 0.6.6" or newer
Initializing a Project
Time to kick off your Agno adventure:
uv init agno-adventure
cd agno-adventure
This whips up a tidy project structure: pyproject.toml
for dependencies, .python-version
(set to 3.12 by default), main.py
to code in, and a README.md
for bragging rights. Lock in Python 3.12 for consistency:
uv python pin 3.12
Setting Up the Environment
Now, create a virtual environment faster than you can blink:
uv venv
source .venv/bin/activate # Windows: .venv\Scripts\activate
Load up the essentials for our AI escapade:
uv add agno lancedb duckduckgo-search openai sqlalchemy psycopg-binary tantivy pypdf pandas
Agno needs an OpenAI API key to flex its muscles, so set it up:
export OPENAI_API_KEY="sk-your-key-here"
Pro Tip: Keep your keys safe in a .env
file with python-dotenv
. Run uv add python-dotenv
, then add OPENAI_API_KEY=your-key-here
to .env
and load it in your script with from dotenv import load_dotenv; load_dotenv()
. Security first, chaos later!
Why uv? It’s not just fast—it’s a one-stop shop replacing pip
, venv
, and more, with a sleek workflow that saves you from dependency nightmares. Think of it as the turbo engine powering your Agno rocket.
Step 2: Your First Agno Agent—Simple, Yet Chatty
Let’s meet Agno, the star of our show. It’s an open-source Python library that makes building AI agents as easy as ordering takeout—but way more fun. Our first agent? A cheerful chatterbox ready to brighten your day.
The Code
Edit main.py
:
from agno.agent import Agent
from agno.models.openai import OpenAIChat
agent = Agent(
model=OpenAIChat(id="gpt-4o"),
description="You’re a cheerful AI pal who loves a good chat!",
markdown=True
)
agent.print_response("Hey! What’s cooking today?", stream=True)
Run it:
uv run python main.py
How It Works
Agent
: The heart of Agno, this class is your agent’s command center, letting you define its personality and powers.OpenAIChat
: Plugs into OpenAI’sgpt-4o
model—think of it as the brain giving your agent its wit. Swap in other models (likegpt-3.5-turbo
) if you’re feeling experimental!description
: Sets the vibe. Here, we’ve got a peppy pal who’s all about good vibes.markdown=True
: Spices up responses with formatting—because plain text is so 2024.print_response
: Streams the reply in real-time, like watching your agent think out loud.
You’ll get a response like, “Hey there! Just here to spice up your day—what’s on the menu?” It’s basic, but it’s alive!
More About Agno
Agno’s lightweight design means it’s nimble yet powerful, perfect for crafting agents that scale from simple chats to complex tasks. Unlike heavier frameworks, it’s built for speed and flexibility, letting you add features like tools and memory without breaking a sweat.
Troubleshooting
- “ModuleNotFoundError”: Forgot a package? Run
uv add agno openai
and try again. - Silent Agent: Check your
OPENAI_API_KEY
. No key, no chat—it’s like forgetting to plug in your coffee maker.
Step 3: Adding DuckDuckGo Tools—Your Web-Surfing Sidekick
Our agent’s charming but clueless about the world. Let’s hook it up with DuckDuckGo tools so it can surf the web like a pro.
The Code
Update main.py
:
from agno.agent import Agent
from agno.models.openai import OpenAIChat
from agno.tools.duckduckgo import DuckDuckGoTools
agent = Agent(
model=OpenAIChat(id="gpt-4o"),
description="You’re a web-savvy AI explorer!",
tools=[DuckDuckGoTools()],
show_tool_calls=True,
markdown=True
)
agent.print_response("What’s the buzz in New York, March 2025?", stream=True)
Run it:
uv run python main.py
How It Works
DuckDuckGoTools
: Equips your agent with a web search superpower. It decides when to use it based on the question—smart, right?show_tool_calls=True
: Lets you peek at when the agent calls its tools, like a behind-the-scenes director’s cut.- Output: Expect something like, “Calling DuckDuckGo… Here’s the latest from NYC in March 2025!” It’s now a worldly conversationalist.
Agno’s Tool Power
Agno’s tool system is modular brilliance. DuckDuckGoTools
is just one option—Agno supports a growing toolbox you can mix and match to suit your needs, from APIs to custom scripts. It’s like giving your agent a utility belt!
Troubleshooting
- No Web Results: Ensure
duckduckgo-search
is in your arsenal—runuv add duckduckgo-search
. - Stuck?: Rate limits might be the culprit. Take a breather and retry. Add
debug_mode=True
to theAgent
for a deeper look at what’s tripping it up.
Step 4: Memory That Sticks—From Forgetful to Faithful
Our agent’s got charisma but forgets everything the moment you blink. Let’s give it a memory upgrade with Agno’s SQLite-backed storage, turning it into a loyal companion.
The Code
Create memory_agent.py
:
from agno.agent import Agent
from agno.models.openai import OpenAIChat
from agno.storage.agent.sqlite import SqliteAgentStorage
from rich.pretty import pprint
agent = Agent(
model=OpenAIChat(id="gpt-4o"),
description="You’re an AI with a memory like an elephant!",
storage=SqliteAgentStorage(table_name="agent_sessions", db_file="tmp/agent_storage.db"),
add_history_to_messages=True,
num_history_responses=3,
session_id="my_chat_session",
markdown=True
)
agent.print_response("I love spicy Thai food. What’s your favorite cuisine?")
pprint([m.model_dump(include={"role", "content"}) for m in agent.memory.messages])
agent.print_response("What did I just say I love?")
pprint([m.model_dump(include={"role", "content"}) for m in agent.memory.messages])
Run it:
uv run python memory_agent.py
How It Works
SqliteAgentStorage
: Saves chat history to a SQLite database (tmp/agent_storage.db
), so your agent remembers even after a reboot.add_history_to_messages=True
: Feeds the last few messages (set bynum_history_responses=3
) into the prompt, giving context.session_id
: Links interactions under one session—use the same ID, and it’s like picking up where you left off.pprint
: Debugging gold—shows what’s in memory after each chat.
Ask about Thai food, then test its recall. It’ll proudly declare, “You love spicy Thai food!” Memory unlocked!
Agno’s Memory Magic
Agno offers multiple memory types:
- Chat History: Every message, stored in
agent.memory.messages
. - User Memories: Specific tidbits (like preferences), persisted via storage.
- Summaries: Condensed versions of long chats for efficiency. This flexibility makes Agno ideal for agents that need to learn and grow with you.
Troubleshooting
- Amnesia: Same
session_id
? Checktmp/
exists (create it withmkdir tmp
if needed). - No Storage: Run
uv add sqlalchemy
—it’s the backbone of SQLite storage.
Pro Tip: For big projects, swap SqliteAgentStorage
for PostgresAgentStorage
via uv add psycopg-binary
. More power, same simplicity!
Step 5: RAG with LanceDB—Knowledge Is Your Superpower
Time to make your agent a Thai cuisine expert with RAG (Retrieval-Augmented Generation). Using LanceDB, it’ll pull recipes from PDFs and back them up with web smarts—interactive style!
The Code
Create rag_agent.py
:
import typer
from rich.prompt import Prompt
from agno.agent import Agent
from agno.models.openai import OpenAIChat
from agno.knowledge.pdf_url import PDFUrlKnowledgeBase
from agno.vectordb.lancedb import LanceDb
from agno.vectordb.search import SearchType
from agno.tools.duckduckgo import DuckDuckGoTools
# LanceDB Vector DB
vector_db = LanceDb(
table_name="recipes",
uri="tmp/lancedb",
search_type=SearchType.hybrid
)
# Knowledge Base
knowledge_base = PDFUrlKnowledgeBase(
urls=["https://agno-public.s3.amazonaws.com/recipes/ThaiRecipes.pdf"],
vector_db=vector_db
)
def lancedb_agent(user: str = "user"):
agent = Agent(
model=OpenAIChat(id="gpt-4o"),
description="You’re a Thai cuisine expert with web backup!",
user_id=user,
knowledge=knowledge_base,
tools=[DuckDuckGoTools()],
instructions=[
"Search the knowledge base for Thai recipes first.",
"Use DuckDuckGo if more info is needed."
],
show_tool_calls=True,
debug_mode=True,
markdown=True
)
print(f"Session ID: {agent.session_id}\n")
while True:
message = Prompt.ask(f"[bold] :sunglasses: {user} [/bold]")
if message in ("exit", "bye"):
break
agent.print_response(message, stream=True)
if __name__ == "__main__":
# Load once, comment out after first run
knowledge_base.load(recreate=True)
typer.run(lancedb_agent)
Run it:
uv run python rag_agent.py
How It Works
LanceDb
: A vector database storing PDF embeddings for lightning-fast retrieval.SearchType.hybrid
blends keyword and semantic searches for max accuracy.PDFUrlKnowledgeBase
: Loads a Thai recipe PDF into the database, fueling RAG—your agent fetches answers from it first.DuckDuckGoTools
: Web fallback when the PDF isn’t enough.typer
/Prompt
: Keeps the chat going until you say “bye”—perfect for recipe hunting!- Output: Ask, “How do I make chicken and galangal coconut soup?” It’ll dig into the PDF, then surf the web if needed.
Agno’s RAG Edge
RAG combines retrieval (from LanceDB) with generation (via gpt-4o
), making your agent a knowledge ninja. Agno’s integration is seamless—add any document, and it’s instantly searchable. Plus, it’s fast enough to keep up with your hunger pangs!
Troubleshooting
- PDF Won’t Load: Verify the URL and
uv add lancedb
. - No Chat Prompt: Add
uv add typer rich
for the interactive goodies. - Slow Start: Comment out
knowledge_base.load(recreate=True)
after the first run—it only needs to build the database once.
Pro Tip: Toss more PDFs into urls
—think cookbooks, travel guides, whatever—to create a custom knowledge empire.
Step 6: Team of Two—Chef and Researcher Duo
Why stop at one agent when you can have a dynamic duo? Let’s pair a Thai chef with a web researcher for a collab that’s pure magic.
The Code
Create team_agent.py
:
from agno.agent import Agent
from agno.models.openai import OpenAIChat
from agno.tools.duckduckgo import DuckDuckGoTools
from agno.knowledge.pdf_url import PDFUrlKnowledgeBase
from agno.vectordb.lancedb import LanceDb, SearchType
from agno.embedder.openai import OpenAIEmbedder
from agno.storage.agent.sqlite import SqliteAgentStorage
# Chef Agent
chef = Agent(
name="ThaiChef",
role="Thai cuisine expert",
model=OpenAIChat(id="gpt-4o"),
knowledge=PDFUrlKnowledgeBase(
urls=["https://agno-public.s3.amazonaws.com/recipes/ThaiRecipes.pdf"],
vector_db=LanceDb(
uri="tmp/lancedb",
table_name="recipes",
search_type=SearchType.hybrid,
embedder=OpenAIEmbedder(id="text-embedding-3-small")
)
),
instructions=["Provide detailed Thai recipes from the knowledge base."],
markdown=True
)
# Researcher Agent
researcher = Agent(
name="WebResearcher",
role="Web info gatherer",
model=OpenAIChat(id="gpt-4o"),
tools=[DuckDuckGoTools()],
instructions=["Search the web for supplementary info when asked."],
show_tool_calls=True,
markdown=True
)
# Team Leader
team = Agent(
team=[chef, researcher],
model=OpenAIChat(id="gpt-4o"),
description="You coordinate a chef and researcher for Thai food queries.",
instructions=[
"Ask ThaiChef for recipes first.",
"If more context is needed, consult WebResearcher.",
"Blend their inputs into a cohesive answer."
],
storage=SqliteAgentStorage(table_name="team_sessions", db_file="tmp/team_storage.db"),
markdown=True
)
chef.knowledge.load() # Comment out after first run
team.print_response("Tell me about Thai chicken soup and its cultural significance.", stream=True)
Run it:
uv run python team_agent.py
How It Works
ThaiChef
: Recipe guru, pulling from the PDF via LanceDB withOpenAIEmbedder
for precise embeddings.WebResearcher
: Web sleuth, digging up cultural context with DuckDuckGo.team
: The maestro, coordinating both agents and weaving their answers together. SQLite storage keeps the team’s memory sharp across sessions.- Output: You’ll get a recipe and a story—like, “This soup’s a Thai staple, tied to ancient herbal traditions!”
Agno’s Team Spirit
Agno’s multi-agent system is a game-changer. Each agent has a role, tools, and knowledge, while the team leader delegates like a pro. It’s lightweight yet robust, designed to scale without bogging down—perfect for complex tasks.
Troubleshooting
- Team Mute: Add
debug_mode=True
to the teamAgent
to spy on the chatter. - Storage Snag: Ensure the
SqliteAgentStorage
import is there andtmp/
exists. - Slow Load: Comment out
chef.knowledge.load()
after the first run.
Pro Tip: Add a third agent—like a spice specialist—to turn your duo into a trio of culinary geniuses.
Conclusion: Your Agno Journey Takes Flight!
You’ve just gone from zero to AI hero! With uv’s blazing speed, you set up a pro environment in seconds. Then, with Agno, you built an agent that chats, surfs, remembers with SQLite, masters RAG with LanceDB, and teams up for epic results. This is Python in 2025—fast, fun, and downright fierce.
Key Wins:
- uv: Your setup’s new best friend—say goodbye to sluggish installs.
- Agno: Lightweight, modular, and speedy, with memory, tools, and RAG that make your agents brilliant.
- Teamwork: Multi-agent collab that tackles big questions with ease.
What’s Next? Dive into Agno’s multimodal tricks (think images or audio), track your agents at agno.com, or—most importantly—whip up that Thai chicken soup your agent’s been raving about. You’ve got the code, the skills, and the laughs—go conquer the AI universe!
Related Posts
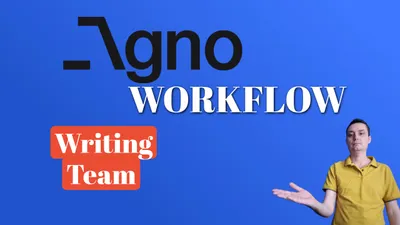
Crafting Beginner-Friendly Tech Articles with Agno Workflows and Streamlit
Discover how to build a powerful AI-driven workflow with Agno to research, outline, write, and edit technical articles tailored for beginners, all wrapped in a sleek Streamlit interface.
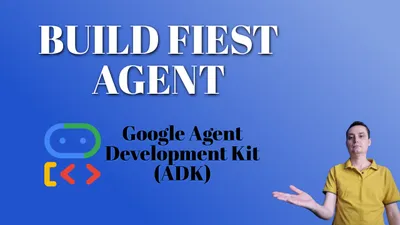
How to Build Your First Agent with Google Agent Development Kit (ADK)
Learn how you can start building your first agent with Google Agent Development Kit (ADK) and add memory and tool use to browse the web.
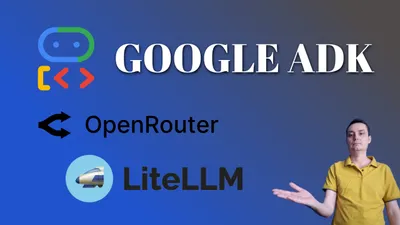
How to Use Any OpenRouter Model with Google Agent Development Kit (ADK)
See how you can use any model you want in Google Agent Development Kit (ADK) with LiteLLM. Configure OpenRouter models easy.